Data type based conversions is a very unique functionality of easy code generator.
It often happens that you need to write code depending on the data type of a database column.
Just imagine you want to write a data class and create properties for all the database columns. Let’s say we have two columns, one is called “databaseProductID” and is defined as NOT NULL column. The other column is called “tableCount” and allows NULL values.
In C# you would declare them like:
private Nullable<System.Int32> databaseProductID;
private System.Int32 tableCount;
or
private System.Int32? databaseProductID;
private System.Int32 tableCount;
In vb.net it would be something like this:
Dim databaseProductID As Nullable(Of Integer)
Dim tableCount As Integer
or
Dim databaseProductID As Integer?
Dim tableCount As Integer
And how would the code look like in other programming languages? You see the dilemma? How should easy code generator know what you want?
We solve this problem with data type based conversions. For each .net data type you can define one parsing code for nullable columns and one for not nullable columns.
Under <Code Snippet Generator> -> <Column Parsing> -> <Data Type Parsing> you can select and change data type parsings.
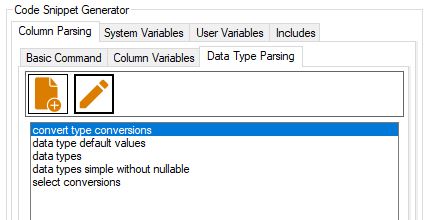
Double clicking on one of the data type parsing groups or pressing the left button, copies the code into your template.
Pressing on the right button, opens the input form for data type parsing groups.
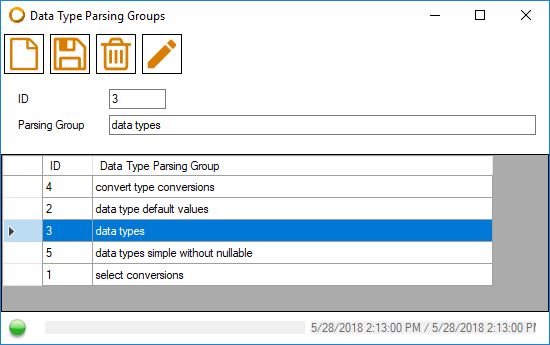
Here you can add, edit or delete your groups.
- ID: primary key, not editable
- Parsing Group: the name of your data type parsing group
Save your changes first before continuing. Now you can open the form to edit the conversion table for this group (click on right button).

If you open this window after adding a new group, this table is pre filled with all available .net data types but the values for the parsing strings are emtpy.
Click on one of the data type in the table and edit the field on top:
- Parsing group ID: primary key, not editable
- Group name: displays the name of the group, not editable
- Parsing string (not nullable): any code that will be replaced if the column is defined as not nullable.
- Parsing string (nullable): any code that will be replaced if the column is defined as nullable.
The parsing strings can be any text, code or even easy code generator commands. For instance, we retrieve data from a database with a data reader (C#) and then assign the value from the reader to a variable. For a datetime data type column the C# code for the parsing strings would look like this:
for non nullable: Convert.ToDateTime(reader[„<<columnName>>“])
for nullable: (reader[„<<columnName>>“] as DateTime?) ?? null
As you see, we use the <<columnName>> tag inside the parsing strings.
Examples
In the examples we again use our table with 4 columns.
1 Variable declaration with default values
To declare variables for all 4 columns of our table, we use the following easy code generator code snippet:
{{<<columnsubstitution>><<crlf=true>><<delimiter=>><<columns=all>>private {{<<dataTypeParsingGroup>><<dataTypeParsingGroupID=3>><<endDataTypeParsingGroup>>}} <<columnName>> = {{<<dataTypeParsingGroup>><<dataTypeParsingGroupID=2>><<endDataTypeParsingGroup>>}};<<endcolumnsubstitution>>}}
Inside the <<columnsubstitution>> command we call two different data type parsings. One with ID=3, followed by the <<columnName>> tag and another with ID=2.
The conversion table for ID=3 you can see in the image above. The conversion for ID=2 defines the default values that we assign during declaration.

After generating our code we get:
private System.Int32 templateGroupID = 0;
private System.String description = „“;
private System.DateTime recordInsertDate = DateTime.Now;
private System.DateTime recordUpdateDate = DateTime.Now;
2 Assign values to variables while reading the data reader
We use a data reader to read data from our table and assign them to the corresponding properties in a data class. We use the following easy code generator code snippet:
{{<<columnsubstitution>><<crlf=true>><<delimiter=>><<columns=all>><<columnNameCap>> = {{<<dataTypeParsingGroup>><<dataTypeParsingGroupID=1>><<endDataTypeParsingGroup>>}};<<endcolumnsubstitution>>}}
Here too we use the <<columnsubstitution>> command to loop through all columns. For each column we assign the reader value to a variable with name <<columnNameCap>>, the column name with the first character capitalized. The data type parsing group returns the complete code to convert the reader value to the correct data type of the column.
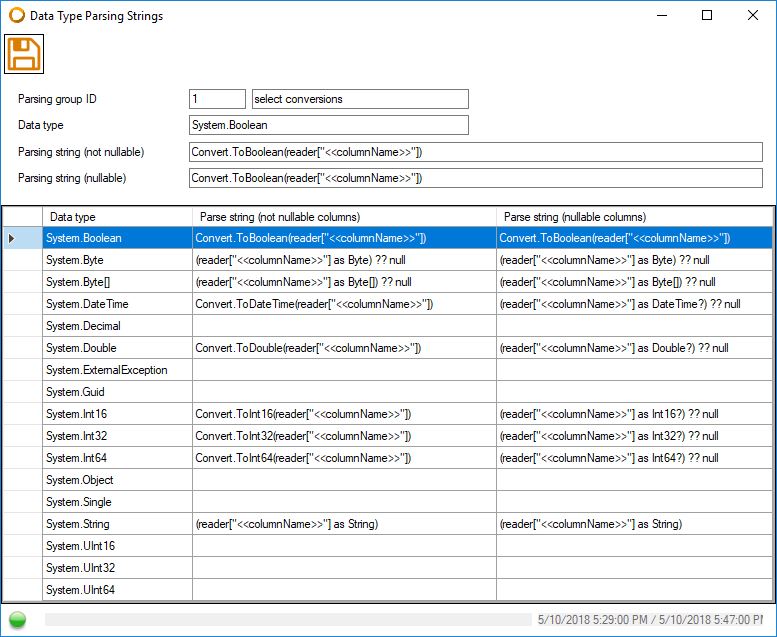
We now get the following result:
TemplateGroupID = Convert.ToInt32(reader[„templateGroupID“]);
Description = (reader[„description“] as String);
RecordInsertDate = Convert.ToDateTime(reader[„recordInsertDate“]);
RecordUpdateDate = Convert.ToDateTime(reader[„recordUpdateDate“]);